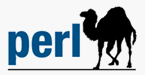
The FORM POST is the most common method of transferring data to a web application. On this post, we will have this implement in PERL which is known to be a back-end processing script.
This is really simple, just follow the 3 simple steps below.
1. Install the Perl Library in your server.
- LWP::UserAgent
2. Initialize user agent and headers.
require LWP::UserAgent;
my $lwpua = LWP::UserAgent->new;
my $user_agent = "Mozilla/5.0 (Windows; U; Windows NT 5.1; en-US; rv:1.8.0.6) Gecko/20060728 Firefox/1.5.0.6";
my @header = ('Referer' => '<domain of where to do post request>',
'User-Agent' => $user_agent);
3. Execute post request with the Parameters in array form and get the return by calling $object->content.
$response = $lwpua->post('<url of where to do post request>',
['<parameter1>' => '<value1>',
'<parameter2>' => '<value2>',
'<parameter..n>' => '<value..n>'], @header);
$return = $response->content;
Hope you like it!! Please see code below for the complete implementation of form post request.
#!/usr/bin/perl
require LWP::UserAgent;
use strict;
use warnings;
my $lwpua = LWP::UserAgent->new;
my $user_agent = "Mozilla/5.0 (Windows; U; Windows NT 5.1; en-US; rv:1.8.0.6) Gecko/20060728 Firefox/1.5.0.6";
my @header = ('Referer' => 'http://domain.to.post.com',
'User-Agent' => $user_agent);
$response = $lwpua->post('http://domain.to.post.com/some.action.page',
['parameter1' => 'value1',
'parameter2' => 'value2',
'parameter3' => 'value3'], @header);
$return = $response->content;
print "$return\n\ndone!";
1;
No comments:
Post a Comment